Hello,
Before I jumped in and started writing a GRIB file adapter,
I thought I'd just try to read one slice of temperature data that
the degrib routine prints out: an binary array of floats,
with a header integer declaring the number of bytes to follow.
I tried looking at the Simple example and the Vis5DForm
code to come up with the following main method.
Also included is a GIF image of the screen output from my program.
It is supposed to be a temperature map (values are in Kelvin).
It looks funky to me! I tried changing the display from RGB
to IsoContour, but I got a NullPointer exception. I printed out
the float values of array, and they look OK. I'm not quite sure
what to test here...
In line 104 in my program, I set a scalarMap using field3d[0]
rather than something from the range I set up earlier like in
the example. Am I accessing the temperature field data as I
thought? I'm not sure how the connection between my FlatField
grid (where I store my array data) and the field3d variable
is made?
If you need the 69K binary data file in order to run it, let me
know and I can send it to you.
Thanks for any insight!
KathyLee Simunich
Argonne National Lab
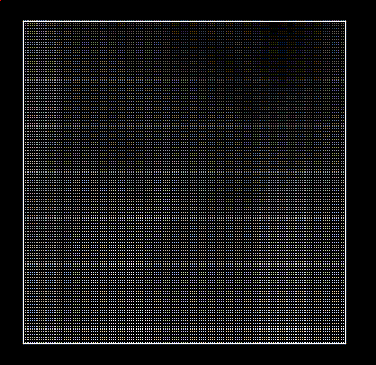
// import needed classes
import visad.*;
import visad.java2d.DisplayImplJ2D;
//import visad.util.VisADSlider;
import java.rmi.RemoteException;
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
import java.io.*;
public class WXView {
public static void main(String args[])
throws VisADException, RemoteException, IOException {
if (args.length < 1) {
System.err.println("Usage: java WXView <filename>");
System.exit(0);
}
float[][] array= null;
String filename = new String(args[0]);
try {
// read in the GRIB slice
RandomAccessFile in = new RandomAccessFile(new
File(filename),"r");
int numVals = WXView.readInteger(in);
int val = numVals/4;
array= new float[1][val];
System.out.println("numVAls= "+numVals+" val = "+val);
// reads in the number of bytes in file
for (int i=0; i<val; i++) {
array[0][i] = WXView.readFloat(in);
}
} catch (FileNotFoundException e) {
e.printStackTrace();
System.exit(0);
}
catch (IOException e) {
e.printStackTrace();
System.exit(0);
}
// construct RealType components for grid coordinates
RealType row = new RealType("row",null,null);
RealType col = new RealType("column",null,null);
RealType level = new RealType("level",null,null);
// construct RealTupleType components for grid coordinates
RealType[] type3d = {row, col, level};
RealTupleType domain = new RealTupleType(type3d);
// construct RealType components for grid fields
RealType temperature = new RealType("temperature",null,null);
// construct RealTupleType for grid fields
RealType[] field3d = {temperature};
RealTupleType range = new RealTupleType(field3d);
// construct FunctionType for grid
FunctionType grid_type = new FunctionType(domain,range);
// construct RealType and RealTupleType for time domain
RealType time = new RealType("time",null,null);
RealTupleType time_type = new RealTupleType(time);
// construct FunctionType for time sequence of grids
FunctionType afwa_type = new FunctionType(time_type,grid_type);
System.out.println(afwa_type);
// construct an integer 3D grid - hardcoded dimensions thus far...KLS
Set grid_set = new Integer3DSet(144,120,1); // what are the numlevs?
// construct a sequence of hours - also hardcoded
Set time_set = new Integer1DSet(1);
// construct a FieldImple for a time sequence of grids
FieldImpl grib = new FieldImpl(afwa_type,time_set);
//loop on time hours...
// construct a FlatField for the ith time step
FlatField grid = new FlatField(grid_type,grid_set);
// set the data values into the grid
grid.setSamples(array);
// set grid as the ith time sample
grib.setSample(0,grid);
// create a Display using Java2D
DisplayImpl display = new DisplayImplJ2D("weather map");
DataReference grid_ref = new DataReferenceImpl("grid");
grid_ref.setData(grib);
// map image coordinates to display coordinates
display.addMap(new ScalarMap((RealType) domain.getComponent(0),
Display.XAxis));
display.addMap(new ScalarMap((RealType) domain.getComponent(1),
Display.YAxis));
// map image brightness values to RGB (default is grey scale)
display.addMap(new ScalarMap((RealType) field3d[0],
Display.RGB));
display.addReference(grid_ref);
// create JFrame (i.e., a window) for display and slider
JFrame frame = new JFrame("Simple VisAD Application");
frame.addWindowListener(new WindowAdapter() {
public void windowClosing(WindowEvent e) {System.exit(0);}
});
// create JPanel in JFrame
JPanel panel = new JPanel();
panel.setLayout(new BoxLayout(panel, BoxLayout.Y_AXIS));
panel.setAlignmentY(JPanel.TOP_ALIGNMENT);
panel.setAlignmentX(JPanel.LEFT_ALIGNMENT);
frame.getContentPane().add(panel);
// add slider and display to JPanel
panel.add(display.getComponent());
// set size of JFrame and make it visible
frame.setSize(500, 600);
frame.setVisible(true);
}
static int readInteger(RandomAccessFile input) throws IOException{
int b1,b2,b3,b4;
b1=input.readUnsignedByte();
b2=input.readUnsignedByte();
b3=input.readUnsignedByte();
b4=input.readUnsignedByte();
//return (int)(b4<<24|b3<<16|b2<<8|b1);
return (int)(b1<<24|b2<<16|b3<<8|b4);
}
static float readFloat(RandomAccessFile input) throws IOException{
return Float.intBitsToFloat(readInteger(input));
}
}